Inspector
Variable inspector
Understanding and using the variable inspector in Wysdom notebooks
The Inspector Panel is a powerful debugging and state inspection tool that helps you analyze your Solidity code in real-time.
Every time you compile a variable, function, contract, struct or library it gets added to the inspector panel to view.
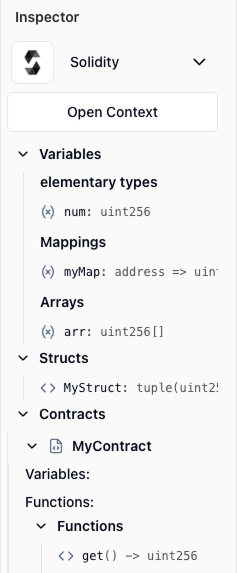
What Gets Added to the Inspector
1. Variables
The inspector automatically tracks:
2. Data Structures
Complex types are displayed hierarchically:
3. Custom Types
User-defined types are fully inspectable:
4. Contracts
When you define a contract, the inspector shows:
- All state variables
- Function list with signatures
- Inherited contracts
- Events and modifiers
5. Libraries
Library functions and their signatures appear in the inspector:
Real-time Updates
The Inspector Panel updates automatically when:
- New code is compiled
- Variables change value
- Functions are called
- State is modified